Table of Contents
- Introduction to React Components
- Class Components
- Syntax and Structure
- Lifecycle Methods
- State and Props
- Event Handling
- Functional Components
- Syntax and Structure
- Props
- State with Hooks
- Side Effects with Hooks
- Class Components vs Functional Components
- Comparison Table
- When to Use Class Components
- When to Use Functional Components
- Best Practices
- Frequently Asked Questions (FAQ)
- Conclusion
Introduction to React Components
React components are self-contained pieces of code that represent a part of the user interface. They can be reused throughout the application, making the code more modular and easier to maintain. Components can receive data through props (short for properties) and manage their own internal state.
React components can be defined in two ways:
1. Class Components
2. Functional Components
Let’s dive into each type of component in detail.
Class Components
Class components are defined using ES6 classes and extend the React.Component
base class. They provide a more feature-rich way of defining components compared to functional components.
Syntax and Structure
Here’s an example of a class component:
import React from 'react';
class MyComponent extends React.Component {
render() {
return <div>Hello, {this.props.name}!</div>;
}
}
export default MyComponent;
In this example, MyComponent
is a class component that renders a div
element with a greeting message. The component receives a name
prop, which is accessed using this.props.name
.
Lifecycle Methods
Class components have access to lifecycle methods, which allow you to hook into different phases of a component’s lifecycle and perform actions accordingly. Some commonly used lifecycle methods include:
componentDidMount()
: Called after the component is mounted to the DOM.componentDidUpdate()
: Called after the component is updated.componentWillUnmount()
: Called before the component is unmounted from the DOM.
Here’s an example that demonstrates the usage of lifecycle methods:
class MyComponent extends React.Component {
componentDidMount() {
console.log('Component mounted');
}
componentDidUpdate(prevProps) {
if (this.props.count !== prevProps.count) {
console.log('Count prop updated');
}
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return <div>Count: {this.props.count}</div>;
}
}
State and Props
Class components can manage their own internal state using the state
object. The state represents the current data of the component and can be updated using the setState()
method. Props, on the other hand, are read-only data passed from a parent component to a child component.
Here’s an example that demonstrates state and props in a class component:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
incrementCount() {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
}
render() {
return (
<div>
<h2>{this.props.title}</h2>
<p>Count: {this.state.count}</p>
<button onClick={() => this.incrementCount()}>Increment</button>
</div>
);
}
}
In this example, the Counter
component manages its own state (count
) and provides a method (incrementCount()
) to update the state. It also receives a title
prop from its parent component.
Event Handling
Class components handle events by defining event handler methods within the component. These methods are typically bound to the component instance using the bind()
method in the constructor.
Here’s an example of event handling in a class component:
class ClickCounter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Click Me</button>
</div>
);
}
}
In this example, the handleClick()
method is bound to the component instance in the constructor. It is then assigned to the onClick
event of the button, so it gets called whenever the button is clicked.
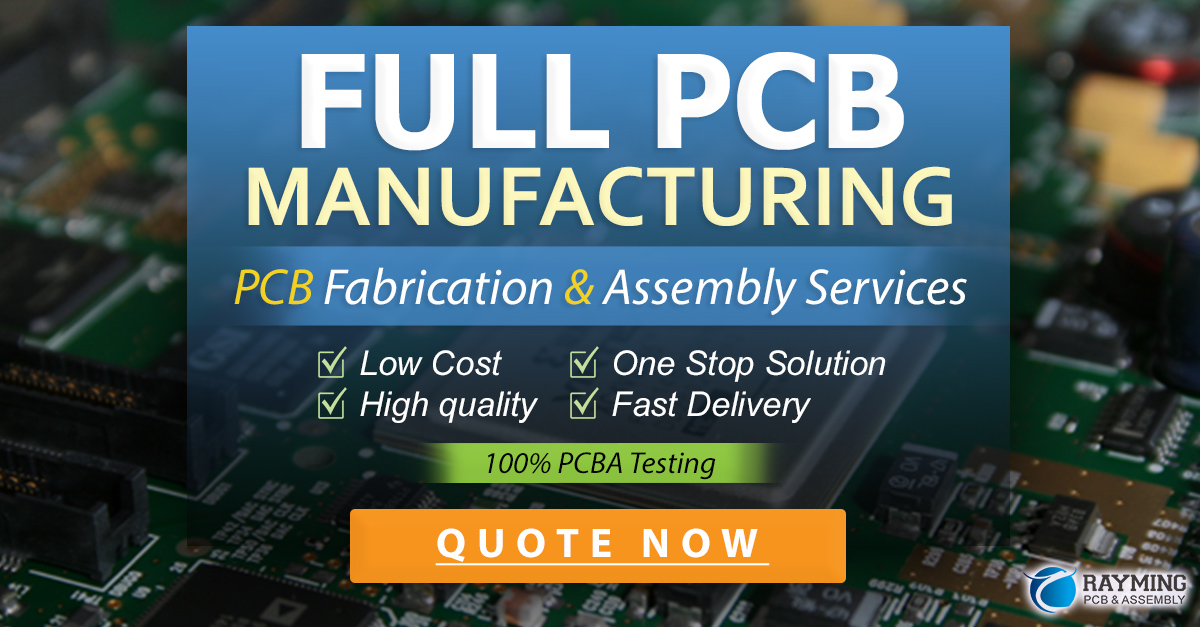
Functional Components
Functional components, also known as stateless components or presentational components, are simpler and more concise compared to class components. They are defined as JavaScript functions that accept props as an argument and return JSX.
Syntax and Structure
Here’s an example of a functional component:
import React from 'react';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
export default MyComponent;
In this example, MyComponent
is a functional component that receives props
as an argument and returns JSX that renders a greeting message.
Props
Functional components receive props as an argument and can use them directly within the component’s JSX.
Here’s an example that demonstrates the usage of props in a functional component:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
In this example, the Greeting
component receives a name
prop and uses it to render a personalized greeting message.
State with Hooks
With the introduction of React Hooks in React 16.8, functional components gained the ability to manage state and lifecycle features. The useState
hook allows functional components to have state.
Here’s an example that demonstrates the usage of useState
hook in a functional component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
function incrementCount() {
setCount(count + 1);
}
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
In this example, the Counter
component uses the useState
hook to manage its state. The useState
hook returns an array with two elements: the current state value (count
) and a function to update the state (setCount
).
Side Effects with Hooks
Functional components can also handle side effects, such as subscribing to events or fetching data, using the useEffect
hook. The useEffect
hook allows you to perform side effects in functional components.
Here’s an example that demonstrates the usage of useEffect
hook:
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState([]);
useEffect(() => {
fetchData();
}, []);
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const jsonData = await response.json();
setData(jsonData);
}
return (
<div>
<h1>Data:</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
In this example, the DataFetcher
component uses the useEffect
hook to fetch data from an API when the component mounts. The fetched data is then stored in the component’s state using the useState
hook and rendered as a list.
Class Components vs Functional Components
Now that we have explored both class components and functional components, let’s compare them side by side.
Comparison Table
Feature | Class Components | Functional Components |
---|---|---|
Syntax | ES6 class syntax | Function syntax |
State | Using this.state and this.setState() |
Using useState hook |
Props | Accessed via this.props |
Received as function arguments |
Lifecycle Methods | Available | Using useEffect hook |
Event Handling | Using this binding |
Using function references |
Performance | Slightly slower | Slightly faster |
Readability and Simplicity | More complex | Simpler and more concise |
When to Use Class Components
Class components are suitable in the following scenarios:
- When you need to manage complex state logic or have multiple pieces of state that depend on each other.
- When you need to use lifecycle methods for specific functionality, such as fetching data or subscribing to events.
- When you are working with older codebases that heavily rely on class components.
When to Use Functional Components
Functional components are preferred in the following situations:
- When you have simple, presentational components that primarily focus on rendering UI based on props.
- When you want to leverage the benefits of React Hooks for state management and side effects.
- When you prioritize code simplicity, readability, and reusability.
- When you are building new applications or components from scratch.
Best Practices
When working with React components, consider the following best practices:
- Keep components small and focused on a single responsibility.
- Use meaningful and descriptive names for components, props, and state variables.
- Avoid excessive nesting of components to maintain readability.
- Use propTypes to define and validate the types of props expected by a component.
- Prefer functional components with hooks for new development, as they offer a more modern and concise approach.
- Use class components when necessary, such as when working with legacy code or complex state management scenarios.
- Regularly refactor and optimize your components to improve performance and maintainability.
Frequently Asked Questions (FAQ)
-
Can functional components have state?
Yes, functional components can have state using theuseState
hook introduced in React 16.8. -
Can class components use hooks?
No, hooks are only available in functional components. Class components cannot use hooks directly. -
Should I always use functional components instead of class components?
It depends on your specific requirements and the complexity of your component. Functional components with hooks are generally recommended for new development, but class components still have their place in certain scenarios. -
How do I convert a class component to a functional component?
To convert a class component to a functional component: - Remove the class declaration and replace it with a function.
- Remove the
render()
method and directly return the JSX. - Replace
this.props
withprops
received as an argument. - Replace
this.state
andthis.setState()
with theuseState
hook. -
Replace lifecycle methods with the
useEffect
hook if necessary. -
Can I mix class components and functional components in the same application?
Yes, you can use both class components and functional components in the same React application. They can interoperate seamlessly.
Conclusion
In this article, we explored the differences between class components and functional components in React. Class components are defined using ES6 classes and provide a more feature-rich way of defining components, including state management and lifecycle methods. Functional components, on the other hand, are simpler and more concise, defined as JavaScript functions that accept props and return JSX.
With the introduction of React Hooks, functional components gained the ability to manage state and handle side effects, making them more powerful and flexible. When deciding between class components and functional components, consider factors such as component complexity, state management requirements, and the need for lifecycle methods.
As a best practice, prefer functional components with hooks for new development, as they offer a more modern and concise approach. However, class components still have their place, especially when working with legacy codebases or complex state management scenarios.
Remember to keep your components focused, reusable, and maintainable by following best practices and regularly refactoring your code.
By understanding the differences between class components and functional components, you can make informed decisions and write efficient and effective React code.
Leave a Reply